If you want to learn how to program here’s a quick suggestion. As a new programming student there are two things you simultaneously have to learn: a programming language like Pascal, C or Java; the second thing is program design concepts. The bad news is that students often prioritize learning the language, paying little to no attention to program design concepts. The student eventually hits a brick wall, gives up, maybe they jump across to a new language where they hit a brick wall again and finally give up, declaring that “programming is too hard”. Learning a programming language without first understanding program design concepts is like learning a foreign language without knowing the rules of grammar and punctuation. Without knowing the rules you can’t write a paragraph because you can’t write a sentence.
Instead focus on program design concepts, the fundamentals, the nuts and bolts. Learn them. Learn them well for these concepts will serve you regardless of the programming language you learn. I’m not at all saying that every programming language suddenly becomes easy. That’s not what I’m saying.
Without ever talking about a programming language, let’s define a program or algorithm (I’m not sure why but I dislike the word algorithm). A program is a series of steps that solve a problem. Exactly how many steps there are depends on the problem. You can put together any programming solution (or algorithm) using three programming constructs: Sequence; Selection; and Iteration/Loops.
A construct is a building block. Like a Lego block. Arrange these blocks in a sensible way and you will build a sensible solution. A quick note before we get to each of the three constructs. I’ve attached diagrams with simple examples of each construct: Sequence, Selection and Iteration/Loops. These diagrams are visual representations of each construct. In each diagram a circle represents: Begin/End; a rectangle represents a single programming instruction like “Ask the user for their name”; and a diamond represents a logical expression (for now let’s pretend a logical expression is a simple question like “Is the age the user entered less than 18?”.
Sequence
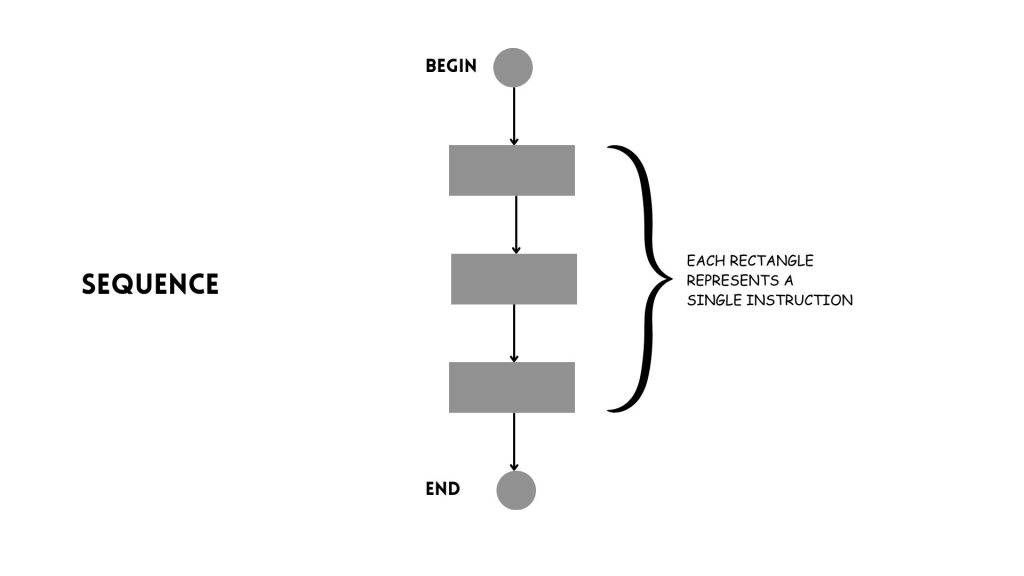
The sequence construct is a series of instructions that happen one after the other: bam-bam-bam! The instructions flow uninterrupted. There is no obstacle. For example, a program begins, the program asks a user for their name and age and the user enters their name and age. Then the program ends.
Selection
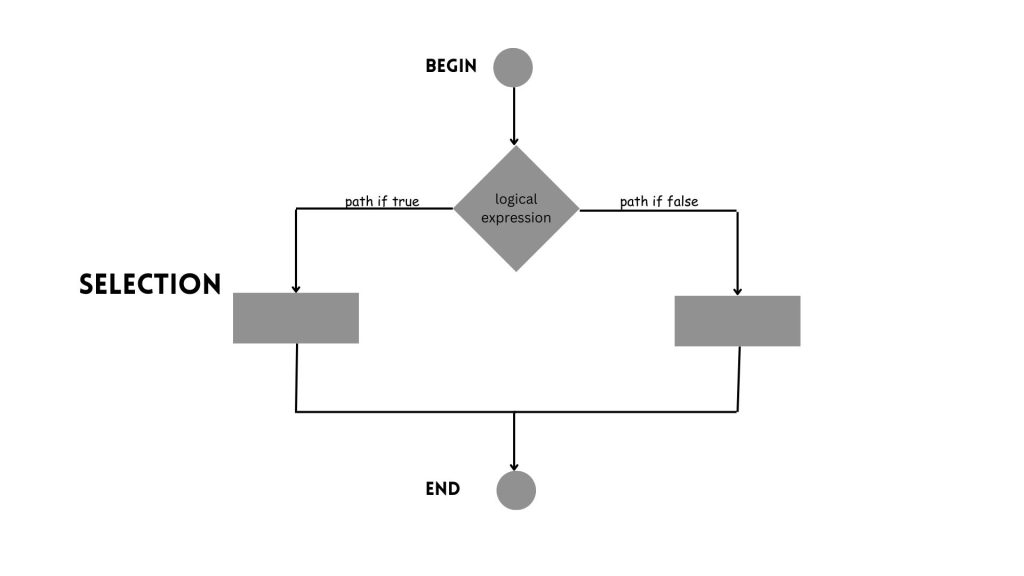
The selection construct. Programs wouldn’t be very useful if they could only perform sequence steps. In life we often have to choose a path: watch a funny video or read a boring programming tutorial. Work out our arms or legs? Arms win! Consider a program that allows a user to vote only if the user is 18 years or older. Else if the user is less than 18, they are NOT allowed to vote. Regardless of the programming language (at least the ones I’m familiar with) the selection construct always asks for a logical expression (we’ll talk about logical expressions another day).
Iteration/Loop
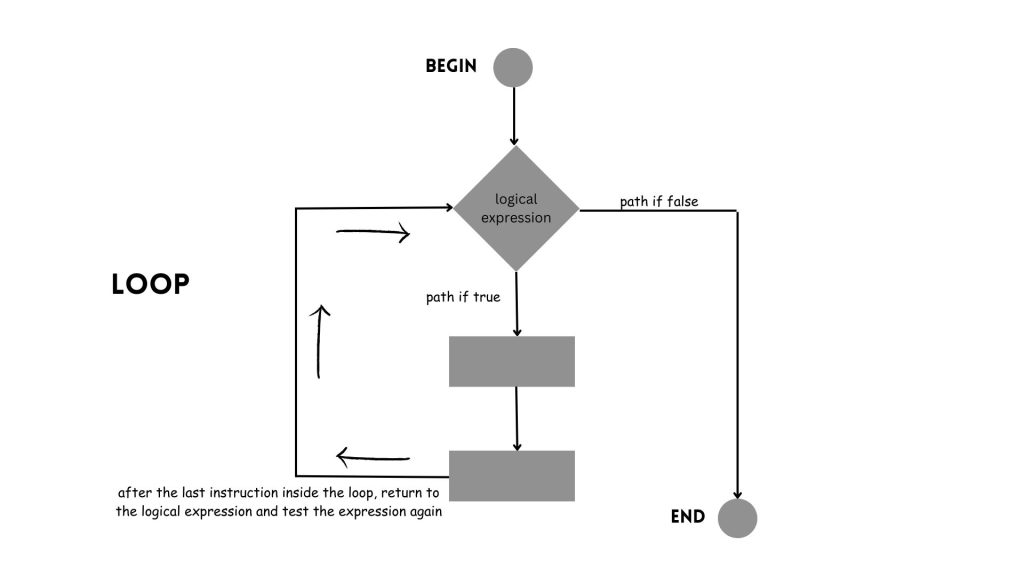
Iteration/Loop construct. Programs or algorithms often have to repeat instructions a certain number of times. The exact number of times is either known or unknown. Consider a real world example where a teacher is in a class with ten students. It’s the first class. The teacher points to the first student, asks for their name, age and hobbies. The first student answers. The teacher points to the second student, repeats the exact instructions, and the second student complies. This happens until the tenth student has said their name, age and hobbies. There isn’t an eleventh student so the introductions end.
Here’s another example of iteration. A grocery cashier is scanning items a customer is placing on a conveyor belt. The cashier isn’t sure how many items are in the cart but each time the cashier scans an item the customer’s bill increases by the cost of the scanned item. This activity continues until there are no more items in the cart. Once the cart is empty, the system displays the total, the customer pays, the transaction ends.
Conclusion
Notice we haven’t talked about any ONE programming language. These three programming constructs are the building blocks of any solution. There are other building blocks like variables, relational operators and expressions that will clarify exactly how sequence, selection and iteration/loops work. Those are lessons for another day. In the meantime, rest assured that, depending on the problem you need to solve, you can write an algorithm/program by stacking these three constructs in different ways. The best part is that you can take your intimate knowledge of these constructs to any programming language.
If you’re interested in my website design course go fill out this form: https://forms.gle/WxiEpFtgpNHhbSe18